110: Data Types: Void And Bool.
Take Up Code - A podcast by Take Up Code: build your own computer games, apps, and robotics with podcasts and live classes
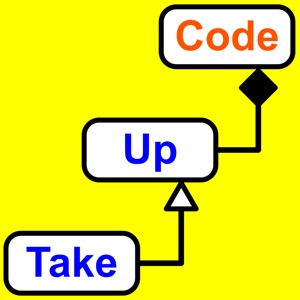
Categories:
Void and bool are simple types that you can use with very little explanation. There are a few details that you should be aware of though. Think of void as an absence of a type and not really a type itself. There are specific places where void can be used and this will depend on your language. Most languages allow you to declare a method that returns nothing by declaring the method returns void. When you do this, then it’s a compile error to try returning an actual value. You cannot use void to declare a variable or a reference but if your language supports pointers like in C++, then you can have a pointer to void. You also cannot use void as a template type in C++ or as a generic type in C#. That’s all for C#. But C++ has one additional twist to using void with templates. In C++, you can specialize a template. Let’s say you have a template that takes two type parameters. You could instantiate an instance of this template with an int and another int. Or a short and a char. Or any two types. But you can’t use void. There is a way to get around this by specializing the template so that the new specialized template only needs one parameter type and the other one is written to use void. So even though you can’t pass void directly through the type arguments to a template, you can use void in a special version of the template. You might expect that a data type that can only hold true or false values requires no further explanation. But there wasn’t always a bool data type available so developers sometimes created their own. And these creations didn’t always match. Most of the time, code that needed to define its own bool data type would define true to be the value 1 and false to be the value 0. A notable exception to this was Visual Basic which defined a type called a VARIANT_BOOL and it used -1 to mean true and 0 to mean false. You can’t just assign true or false to your variable but will need to use special VARIANT_TRUE and VARIANT_FALSE values that have been defined to be -1 and 0. Listen to the full episode about void and bool types, or you can also read the full transcript below. Transcript Episode 7 explains the various data types including void and bool but it was mainly an overview. This episode goes into more detail about void and bool. Alright, think of void as an absence of a type and not really a type itself. There are specific places where void can be used and this will depend on your language. Most languages allow you to declare a method that returns nothing by declaring the method returns void. When you do this, then it’s a compile error to try returning an actual value. You have two options. The first is to just let the method end without returning anything. This means you just don’t put a return statement at the end of your method. The second option is used when you want the method to return early and in this case, you just write a return statement. Many return statements have a variable or some other value after the return statement. But when you have a return with nothing after it, that allows you to return from a method at that point without returning anything to the caller. Some languages like the C language use void in the parameters of a method to mean the method requires no arguments to call the method. Other languages like C++ and C# just use empty parenthesis after the method name to mean there are no parameters. You cannot use void to declare a variable or a reference but if your language supports pointers like in C++, then you can have a pointer to void. This is useful especially for methods that allocate memory where the memory will be used for some unknown purpose. At least the method that allocates the memory has no idea what the memory will be used for. If you call such a method to get some memory, then you should have some purpose in mind for the memory. Once you get the memory, then you can cast the void pointer to a pointer to a specific t