127: Data Types: Smart Pointers Part 2.
Take Up Code - A podcast by Take Up Code: build your own computer games, apps, and robotics with podcasts and live classes
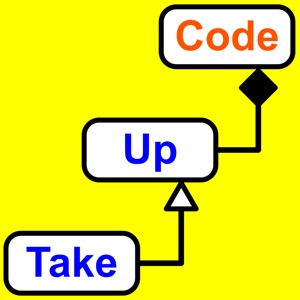
Categories:
The C++ language guarantees that destructors run at specific times even if an exception is thrown. You can use this to make sure that other cleanup work gets done. That’s what smart pointers do. A pointer can allow you to create new objects in some scope and then pass that object outside the scope that it was created in. What I mean is that you can have a method with all its local variables existing on the stack that will get destroyed when the method completes. But this method can allocate a new object on the heap, get a pointer to that new object, and pass that pointer back to the calling code. Ownership of the newly created object now belongs to the code that called the method and what it does with that pointer is up to that code. Maybe it sends the pointer and therefore ownership of the pointer to yet another method. It’s really important to have a clear understanding of what code is responsible for that pointer. And that’s what this episode will explain. There are times when you want to make sure that only a single owner exists for a pointer. Just imagine the problems if both you and your neighbor thought you each owned the same car. In order to prevent this, C++ has a unique_ptr class that makes sure only a single piece of code owns a pointer at any time. You can transfer ownership but can’t have multiple owners. And there are times when you do want multiple owners. In this case, you want to make sure that the owned object stays around until the last piece of code is done with it. This is like a shared driveway that you and your neighbor both maintain. Just because you decide that you don’t need a car anymore doesn’t mean that you can delete the driveway and plant vegetables there. The driveway needs to remain as long as either of you need it. C++ has a shared_ptr class to help with this situation. Listen for more information about these as well as another type, a weak_ptr, that works with the shared_ptr. You can also read more in the full transcript below. Transcript This episode continues explaining smart pointers and how to use the smart pointers in the C++ standard library. There’s two main concepts to understand, exclusive ownership and shared ownership. But why is ownership an issue at all? A pointer can allow you to create new objects in some scope and then pass that object outside the scope that it was created in. What I mean is that you can have a method with all its local variables existing on the stack that will get destroyed when the method completes. But this method can allocate a new object on the heap, get a pointer to that new object, and pass that pointer back to the calling code. Ownership of the newly created object now belongs to the code that called the method and what it does with that pointer is up to that code. Maybe it sends the pointer and therefore ownership of the pointer to yet another method. It’s really important to have a clear understanding of what code is responsible for that pointer. And that’s what this episode will explain. There are times when you want to make sure that only a single owner exists for a pointer. Just imagine the problems if both you and your neighbor thought you each owned the same car. In order to prevent this, C++ has a unique_ptr class that makes sure only a single piece of code owns a pointer at any time. You can transfer ownership but can’t have multiple owners. And there are times when you do want multiple owners. In this case, you want to make sure that the owned object stays around until the last piece of code is done with it. This is like a shared driveway that you and your neighbor both maintain. Just because you decide that you don’t need a car anymore doesn’t mean that you can delete the driveway and plant vegetables there. The driveway needs to remain as long as either of you need it. C++ has a shared_ptr class to help with this situation. Let’s look at the exclusive ownership