21: C++ Creating And Deleting Instances.
Take Up Code - A podcast by Take Up Code: build your own computer games, apps, and robotics with podcasts and live classes
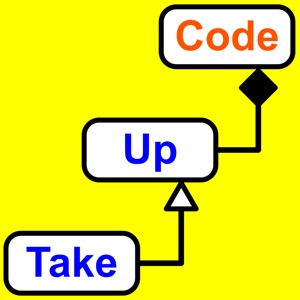
Categories:
You’re ready now to apply what you’ve learned about class definitions, pointers, and constructors and destructors to be able to create new instances in the main computer memory. There are some fundamental differences between C++ and C# that we’re going to again focus on just C++ first and then explore C# in tomorrow’s episode. You learn what an instance of your class is, where it lives in memory, how small can it actually be, and how to dynamically allocate the memory to create instances and then delete them when you’re done. Along the way, we’ll explore the stack again. You’ll learn a bit more about global variables and something new called static variables. And you’ll learn when and how to use the heap to store your variables. I also explain how the C++ runtime interacts with your operating system to manage memory. You can listen to the full episode or read the full transcript below. Transcript The basic structure of your class data is defined by what data members you included in your class definition. You’ll usually find class definitions in header files which get included by your source files. The source files will normally contain the implementation of the class methods. When you put the methods together with the data, you get the fundamental concept of object-oriented programming. But all this is just a definition. Think of it like a stencil that you can use to paint images on walls. One stencil can be used over and over to create multiple image instances. And the images can even use different colors. The stencil just defines the basic shape and size of the images. Classes and structs are like these stencils. They define what data and methods make up the class instances. Any particular instance can have its own values for the data that the class defines will exist. For example, a class can declare that it has a data member to hold a color. But you need an actual instance of that class in order to get a specific color. The class says that there will be a color and the instance says what the color will be. You could have hundreds of instances of this class each with a slightly different color. But they all have a color. What if a class has no data? Is this allowed? For that matter, does a class need methods? It’s possible to define a completely empty class with no methods and no data. The compiler will cheat a bit here and will give you a few methods anyway. Anytime you have no constructor declared, you’ll get a default constructor. You’ll also get a basic copy constructor and a destructor. So you have this empty class that’s not so empty after all but it still defines no data members. How much memory will be required for an instance of this class? In other words, how much wall space will this stencil use when painted? There’s a rule in C++ that the smallest size anything can be is one byte. So even an empty class will require a single byte even if nothing will ever be written to that byte. Notice that I’m not talking about the size of the instructions that make up the class methods. An instance of a class consists of its data. When we get to inheritance, you’ll also learn about virtual functions. If a class has any virtual functions, then its instances will be a little bigger. The term function is really just another word for a method. At least in C++. Some languages might have different concepts or make some distinction between a method and a function. And some languages may prefer one term or another. I tend to use them interchangeably. So why can an instance be no smaller than a byte? C++ is such an efficient language, why start wasting bytes now? It’s because when you create an instance, that instance needs to live somewhere in memory and must have a memory address that is unique to just that instance. It’s like walking into a restaurant that has a maximum occupancy of one. You’ll have the whole restauran