23: Access Control. Employees Only.
Take Up Code - A podcast by Take Up Code: build your own computer games, apps, and robotics with podcasts and live classes
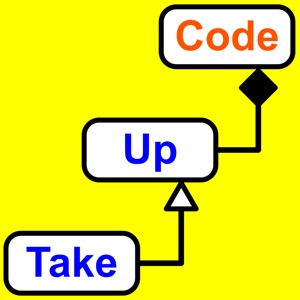
Categories:
When defining what data and methods belong to a class, you also get to set accessibility levels so that some things are available for general use while other members are more restricted. Why not just make everything available? Then you don’t have to worry about it, right? Learning proper access control will help you write code that’s actually easier to understand and easier to use. This episode will explain why. The access levels discussed that apply to both C++ and C# are: public – Makes members available to any code in the application. protected – Makes members available only to classes that derive from your class. private – Allows only the class itself to have access. And the access levels that apply only to C# are: internal – Makes members available only to code within the same assembly. protected internal – Makes members available only to code within the same assembly or to any derived class either in the same assembly or not. If you want a starter class that you can use to create all of your classes, just go to takeupcode.com/offers where you’ll find links to all our special offers. Listen to the full episode or read the full transcript below. Transcript Picture yourself in a small clothing store that has an area in front to display merchandise. You’re looking around when you see a door with a sign that says “Private: Employees Only.” That sign is there to let you know what parts of the store you should not visit. Employees of the store are allowed to enter but the general public really has no business in that part of the store. Imagine if this concept didn’t exist and you’re browsing the store when you see an interesting shirt with no price tag. You take the shirt to the counter only to find out that it’s not for sale because it needs some modification first. This isn’t the best shopping experience and it gets worse. You take the shirt back and can’t remember exactly where you found it so you put it down on another table. Later when the owner of the store arrives, he can’t find the shirt he was working on the day before. Keeping things private not only helps the consumer, it also helps the employees. And the same concept applies to object-oriented programming. When you create a class, you’ll need to specify which methods are public. You can also make data members public but I don’t recommend that. Instead of making a data member public, keep it private instead and create methods that get and set the data value. These methods are called getters and setters. If you only want consumers to be able to read the data, then either don’t create a setter or keep the setter private. If you don’t specify anything for public or private, then by default C++ and C# classes start out as private. If everything’s private, then your class won’t be much use to anybody. In C++, this default level is really the only difference between classes and structs. Classes start out private until you specify otherwise and structs start out as public until you specify otherwise. There’re some other differences between languages that I’ll explain in just a moment. When I say that you’re creating a class, I’m not talking about creating instances of your class in code. I’m talking about creating a new source file and header file, if applicable, and writing the definition of the new class. You’ll need to give the class a name, declare methods and data members, and figure out which methods should be public. Creating a class means that you’re writing the definition and code that make up the class. You can do some interesting things with accessibility levels. For example, if you make all your class constructors private, then no other code will be able to create instances of your class. What good is that you might wonder? Well, you could then create a public method that other code c