25: Inheritance. Overriding Methods.
Take Up Code - A podcast by Take Up Code: build your own computer games, apps, and robotics with podcasts and live classes
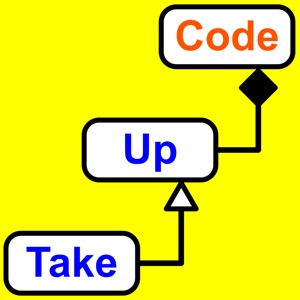
Categories:
Declaring that a class is another class type is only part of the reason to use inheritance. It’s actually much more powerful than just allowing you to refer to a group of different class instances all by some common base type. When you create a new class that derives from a base class, you can actually change the behavior of the base class methods to adapt them to the specific needs of the new derived class. You start this process in a base class by declaring a method to be virtual. Just use the keyword virtual before the method declaration in the class. A very important point that you must remember is that if you intend a class to serve as a base class, then make sure to declare the destructor to be virtual. The end of this episode explains how your classes may not be properly destructed if you forget this. This episode describes a specific example of a class called bee and another class called bumbleBee. Both classes declare a method called sting and they each have slightly different behavior. It’s this difference in behavior that you should be on the lookout for when deciding if you need to inherit from another class. Listen to the full episode or read the full transcript below. Transcript This is called overriding a method. The process starts in the base class. Whenever you’re designing a class, you should ask yourself if your class will ever need to serve as a base class for some other class. If so, then you can facilitate this by thinking about which of your class methods some other derived class might want to change. You then declare these methods to be virtual. One very important point is that in order for a class to work properly as a base class, you must declare your class destructor to be virtual. Even if you have no other methods that you think a derived class might want to override, make sure that your destructor is virtual. If you don’t do this, then it’s a huge sign that maybe your class should not be derived from at all. So make sure that you declare at least a virtual destructor if you think there’s any chance that some developer will ever want to create a class that derives from your class. You declare a method to be virtual by putting the keyword virtual in front of the method declaration in your class declaration in the header file. At least for C++ that has header files. C# also uses the virtual keyword just without a separate .cpp file for the method implementations. So in C++, if you then implement the virtual method in a .cpp file that’s outside of your class declaration, then you don’t use the virtual keyword there. The virtual keyword goes only in the class declaration. Declaring any method in your class to be virtual makes your instances slightly larger in memory. But you only pay for this with the first virtual method. Adding more virtual methods will not add any extra memory requirements. There’s a little more work that needs to be done to call a virtual method. It basically means that whenever you call a virtual method, you end up going through another layer of code that’s really just a pointer to the actual method that will be called. This extra layer is called a vtable which stands for virtual table. The table is just a series of pointers that point to the most derived method for your class. What does this mean? Let’s start out with a class that doesn’t inherit from any other class. This will be our base class for this example. Let’s give it the witty name bee. Most programming examples use a boring letter B but we’re going to use bee as in the insect that flies and makes honey. Our bee class will have a method called sting and we’ll declare this method to be virtual so that other classes can change it if needed. And let’s not forget to make the destructor virtual. When we implement the sting method for the bee class, it will eventually cause the instance of the bee to die because in real life, bees