31: Getters And Setters Keep It Together.
Take Up Code - A podcast by Take Up Code: build your own computer games, apps, and robotics with podcasts and live classes
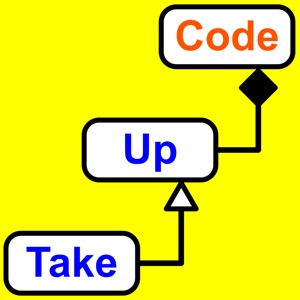
Categories:
Your classes will likely have data members so how do you work with these data members? Should they be public? Private? Do you let other code access them directly? And what’s the difference between a field and a property? This episode will help you get and set your data members. I recommend that you keep your data members private and to create getter and setter methods if you’re using C++ and to create properties if you’re using C#. Even if you don’t think you’ll need the methods or properties right away, they give you so much more control over how your class is used and almost all classes will eventually want this control. There’s just too many ways that outside code can misuse direct access to your class data. And if that’s not enough of a reason, then consider the benefits of testing. Methods and properties help to reduce the exposed surface area of your class and provide well known entry points which can be better tested. Any problem found can then be fixed in one place instead of then trying to figure out where are all the other places in your code where you were working directly with the data. Listen to the episode or read the full transcript below. Transcript Classes allow you to combine data with methods. That doesn’t mean that your data has to be read or written directly. You have options. Your first option is to just make the data available publicly. Let’s say we have our adventure hero class that has an integer value called health. If some other code wants to work with the hero class, it will declare a variable of type hero, give it a name, let’s say, mainHero, and then it can read and write the health value directly with mainHero.health. This is simple and direct. If you don’t want outside code to be able to access the health value directly, then you can just make it private or protected. That’s sort of an all or nothing approach but you can do better. Before we talk about making the design better, what’s wrong with just exposing the health value publicly? Imagine that your game has health potions that restore health and these potions come in different sizes. If some outside code has direct access to the health value, then it might try applying a large health potion that restores 100 points of health. But if your hero was only slightly injured, then letting outside code add 100 points could bring your hero far above his maximum health. And a similar problem can happen in the other direction. If your hero is low on health with only 5 points remaining and takes 10 points of damage, this should cause your hero to die and the game end. But what if the outside code only knows to subtract 10 points and didn’t realize that it also needs to check the remaining health to see if the hero is still alive or not? What you really need is the ability to run some of your own hero class code anytime the health value needs to change. And maybe even when the health value is read too. If you keep the data private, then your second option is to provide methods for reading and writing the value. The methods will have access to the private data because they’re class members. The method to read a value is called a getter and it normally requires no parameters, returns the value, and will be named after the value it returns. Many implementations are trivial and just have a single line of code that returns the private value. But you can do whatever you want including calculate the value if you have no direct value to return. You probably don’t want to change the value before returning it because most callers would find that unexpected. The method that writes a value is called a setter and it normally requires a single parameter with the new value, returns void, and is also named after the value it changes. The implementation of this method can range from trivial to complex depending on what you want to do with the new value. At a minimum, youR