45: Collections: Iterators Part 1.
Take Up Code - A podcast by Take Up Code: build your own computer games, apps, and robotics with podcasts and live classes
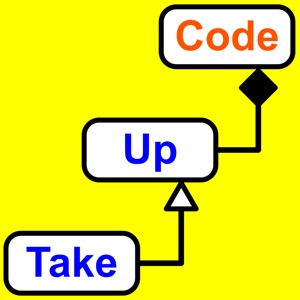
Categories:
Iterators give you the ability to navigate from one item to another in a collection. Why is this so special? Now that you know how to work with various collections, you know that they’re structured very differently. An array just needs to move a pointer to the next item. A list needs to follow wherever the next pointer leads. A binary tree needs to go up and down the tree. Iterators give you a common way to navigate no matter what kind of collection you’re using. If you have a collection like this:list<int> myNumbers;Then you can access the first number in the list like this:auto beginIter = myNumbers.begin();And you can access the end iterator like this:auto endIter = myNumbers.end();Then you can create a for loop to process items in the list like this:for (; beginIter != endIter; ++beginIter) { // Add your code here. }Listen to the full episode about iterators, or you can also read the full transcript below. Transcript Iterators in effect give you a way of not just navigating from one item to another but also of representing a specific location in a collection. Since there are different kinds of collections, that means there are different kinds of iterators. So how do you know which iterator to use? The really nice thing is that you don’t have to worry about this. You just ask whatever collection you happen to be using for an iterator and let it figure out how to give you the correct iterator. This way of thinking is something you grow into as you become more comfortable with object-oriented programming. Let the objects themselves determine how they behave and how they’re related to other types. This is why I urge you to learn an object oriented language first. It’s sort of like how you’re most comfortable with your first spoken language. It takes a lot of work to become as comfortable with another spoken language. The same thing applies to programming. If you learn a procedural language first such as the C language, then you’re naturally going to approach problems with that mindset. Asking a collection for an iterator and not needing to worry about what type of iterator you get is just the beginning though. Let me give you an example. Have you ever tried to count while somebody else was also counting out loud? Maybe the other person was just trying to confuse you and counting randomly or backwards. Many of us get our counting confused. At the very least we have to put extra concentration into our own counting, right? The same thing happens with programming. Maybe you have two collections and one is a list while the other is a binary tree. You want to start processing one item at a time from each collection. Without iterators, this would be a chore because you’d have to write code to navigate two different structures and keep them separate. With iterators though, you just ask for an iterator from each collection and let each one track its own progress as you move from one item to the next. But it gets even better than that. You don’t have to ask for just one iterator from a collection. Maybe you need to pause for a while at one item while continuing on at the same time. You can ask for two iterators and use each one to navigate items in the collection independently of the other iterator. If you know about range-based for loops, then you might be wondering why you need iterators. A range-based for loop usually reads something like this: ◦ “for each object called inventory in the backpack collection perform this block of code” Using a range-based for loop really is the preferred way to write your code if you want to run some code for every item in the collection. It establishes your intent better than a for loop and iterators. But if you don’t want to process all the items, or maybe you need to save an iterator to a particular item so you can come back to it later, then a range-based for loop no longer fits. A range-based for loop actually us