47: Operators. Who Goes First?
Take Up Code - A podcast by Take Up Code: build your own computer games, apps, and robotics with podcasts and live classes
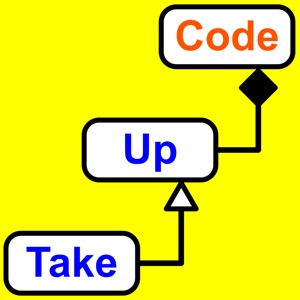
Categories:
Programming languages have a lot of operators. More than most calculators anyway. Do you know what they are? Did you know there’s an order to them? This episode explains precedence, associativity, and evaluation order. Let’s say you have this statement: int i = 2 + 3 * 4 – 5; The multiplication takes precedence over the addition and subtraction and goes first. The multiplication operator uses the symbol * and is a binary operator. This means it takes two operands. In this case, that is 3 and 4. After multiplying 3 by 4, it’s as if the statement now becomes: int i = 2 + 12 – 5; Both the addition and subtraction operators have the same precedence but they have left-to-right associativity which means that the addition is performed next. And then the subtraction. If you want to change this order, you can use parenthesis. So if you wanted 2 to be added with 3 first, then you could write your code like this: int i = (2 + 3) * 4 – 5; You also have unary operators that require only a single operand like this pointer dereference: int i = 5; int * iPtr = &i; int iCopy = *iPtr; Notice how the same asterisk symbol is used to mean multiplication above, then used here to declare a pointer type and then used again to dereference the iPtr pointer and assign the value 5 to the iCopy variable. Many languages use symbols for different purposes and they really mean different things. The meaning will be clear form the usage. It has to be or the compiler won’t be able to understand your code. You can also write the first example to use methods instead of integer values. Then it’ll look like this: int i = methodA() + methodB() * methodC() – methodD(); Here, I’ve just replaced 2, 3, 4, and 5 with method calls. You already know that the multiplication will be done first before the addition or subtraction. But what order will the methods themselves be called? In other words, will the compiler choose to call methodB before or after calling methodC? It has to call both methods before it can perform the multiplication and that’s all you can rely on. The actual order is up to the compiler implementation and could change from one version of your compiler to the next. Listen to the full episode for more about operators, or you can read the full transcript below. Transcript Let’s say you have a local integer variable that you want to assign a value like this: ◦ int i = 2 + 3 * 4 – 5; This is called a compound expression because there’s more than one operator involved. First there’s the assignment operator. Now, I don’t count this operator when classifying something as a compound expression or not. At least that makes sense to me. But I could be wrong about that. The important thing I wanted to explain is the part after the assignment operator. I’m going to take a short detour here to explain operators and how many operands they need. Then I’ll come back to the compound expression. In C++ and in many other languages, you have unary, binary, and ternary operators. A unary operator takes a single operand. The pointer dereference operator is a good example of a unary operator. It looks just like the asterisk used in multiplication but when applied to a pointer, it means to take the value that the pointer points to. It just needs a single pointer variable to work. A binary operator requires two operands. The addition, multiplication, and subtraction operators, plus, asterisk, and minus, are all binary because they need two values to work. You can’t multiply just a single number. Notice how the asterisk symbol is used for both dereferencing pointers and for multiplying numbers. This happens sometimes and the meaning of the symbol needs to be understood from how it’s being used. There’s a single ternary operator that needs three operands. This is the conditional operator and uses two symbols between the three operands. It expects the first ope