49: C++ Preprocessor. This Is Old Magic.
Take Up Code - A podcast by Take Up Code: build your own computer games, apps, and robotics with podcasts and live classes
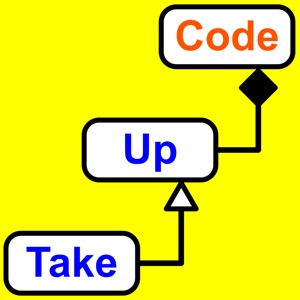
Categories:
The preprocessor is old, primitive, and strong. But you need to understand its strengths to use it effectively. It adds capabilities that the C++ compiler can’t come close to duplicating on its own. All the preprocessor directives begin with the hash character, #, and do not use a semicolon at the end. The end of a directive is just the end of the line. You can extend a preprocessor directive to other lines by ending each line to be extended with a backslash character, \. The using statement is the most common preprocessor directive and might look like this:#include <iostream> #include "../OtherProject/CustomClass.h"You’ll also often find conditional sections like this:#ifndef __cplusplus #error You must compile this code with a C++ compiler #endifA very common pragma directive that allows you to specify that an include file should be processed only once no matter how many times it gets included in a particular compilation unit is:#pragma onceAnd you can also use preprocessor directives to define elaborate replacement text like this:#define MYCLASS(name, id) class name ## id { };Using this macro like this:MYCLASS(MyClass, A)Would be equivalent to writing the following:class MyClassA { };This macro not only replaced MYCLASS with the content of the macro but the ## symbols caused the text passed as name and id to be concatenated to form the class name. Listen to the full episode, or you can also read the full transcript below. Transcript It’s called a preprocessor because it runs first before the compiler ever starts working with your code. You use it all the time without even realizing it. Anytime you write #include and then the name of some standard header file or one of your own header files, then you’re using the preprocessor. I’m going to explain seven aspects or capabilities of the preprocessor with some examples. All the preprocessor directives begins with the hash character. The preprocessor also doesn’t use semicolons to mark the end of a directive. A preprocessor directive goes all the way to the end of a line. The end of a line also marks the end of a preprocessor directive but you can if you want extend very long directives onto the subsequent lines by ending each line to be continued with a backslash character. Number one is the most common and one that I just mentioned #include directives are followed by the name and optional path to a header file. The header file is placed within angle brackets for standard header files that come with the C++ compiler itself or that come with various libraries that you can install. These directives cause the contents of the header file to be included in the file being compiled. If the included file has its own #include statements, then they’re also included. You use header files to define the basic structure of classes or to declare information such as method declarations to be used elsewhere. Putting this information in header files allows you to define it once and make use of it wherever needed without having to re-declare everything each time. Think of this like a rubber stamp that you can use to imprint text over and over again in documents. Number two is also used quite regularly whenever you want to selectively include or exclude sections of your code. These are conditional directives and you have the following available: ◦ #if, #ifdef, #ifndef, #else, #elif and #endif ◦ Some of these are abbreviated in code but when speaking their names, I’ve always found it easier to pronounce them fully. For example, #elseif is how I pronounce #elif. To me, else if just sounds better than elif. Just like how we normally write st in addresses but say street when talking. ◦ These directives work together to test conditions and then either include or exclude entire sections of code. You can also have other preprocessor directives inside these sections that get included or excluded. ◦ You can use these to change how your code is writte