77: Design Patterns: Observer.
Take Up Code - A podcast by Take Up Code: build your own computer games, apps, and robotics with podcasts and live classes
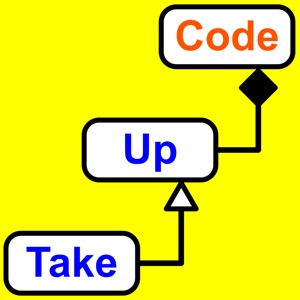
Categories:
What is the observer design pattern? The observer behavioral pattern allows you to be notified of any changes instead of constantly checking. Publish/subscribe is another name that better describes how one part of your code can subscribe to changes while another part only worries about notifying subscribers when something changes. You’ll almost certainly have many different objects in your application that all use some common information. If this is a financial application, then you might have some daily balances that you need to show to the user. A good application will show the balances in multiple ways maybe in a table where they can be changed and in a chart where they can be viewed graphically. If this is an adventure game, then the hero will have a position and a direction. There will usually be a main view that shows the environment that the hero is looking at. And there might be a miniature map in the corner that shows a dot for the position and rotates to show the direction. In each of these cases, you could make one of the views dependent on the other. The chart could constantly ask the the table if anything has changed. It would have to do this rapidly over and over. The miniature map could constantly ask the main view for the position and direction over and over. Or maybe instead you decide to have the table inform the chart whenever it changes. And the main adventure view could notify the miniature map anytime the hero moves or turns. But what do you do when the user wants a different type of chart? Or even worse, how do you handle the case where the user zooms in on the miniature map and makes it go full screen so there’s no more main view? The solution is to refactor the data into its own class that’s not responsible for any of the views. Then any class that is interested to know when the data changes can call a method on the data class to attach a callback method which the data class will call. This method will be called update. The data class defines what the update method looks like because it’ll be the one calling the method. The data class actually just calls however many update methods were attached one at a time until it’s called all of them. Once this is done, each of the views become equals and are no longer dependent on each other. They are each waiting to have their update method called. The episode describes more details and gives some other design considerations you should be aware of. If you’d like to read the book that describes this pattern along with diagrams and sample code, then you can find Design Patterns at the Resources page. You can find all my favorite books and resources at this page to help you create better software designs. Listen to the full episode or you can also read the full transcript below. Transcript The basic description says that this pattern defines a one-to-many relationship between objects so that when an object changes, all dependent objects are notified and can update themselves. This description is a little hard to understand, so let me explain. I’m sure you’ve heard the stories of parents taking their kids on vacation and getting endless questions, “Are we there yet?” Over and over. You might think we’d outgrow this but evidently not when it comes to software. In many ways, even the name of this pattern is wrong. Just think what it means to observe something. Really observe it. Your whole attention is devoted to watching and examining this thing. That’s not what this pattern is about at all. Another common name for this pattern is publish/subscribe which is better. At least when you subscribe to a magazine, you don’t have to observe your local newsstand each day to know when the next edition has been published. It just shows up in your mailbox. Even though the name might be a bit misleading, there’s nothing wrong with this pattern itself. It’s actually very important to get thi