86: Design Patterns: Component.
Take Up Code - A podcast by Take Up Code: build your own computer games, apps, and robotics with podcasts and live classes
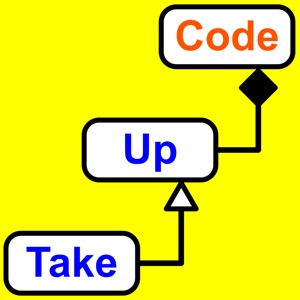
Categories:
What is the component design pattern? The component behavioral pattern allows you to add just the features to your objects that are needed and keep the features independent of each other. The episode describes an example of how best to deal with an adventure game hero class. It gets very confusing if you try to make a particular game class, such as the hero, directly handle all the code needed to process input, detect collisions, update locations, and render images. A better solution is to put each of these operation into their own class. The first step then is to separate these functional parts, or features, or domains, into their own classes. To bring the behavior back into the hero class, you could try to use inheritance but this leads to a rigid solution that’s defined at compile time. This episode explains how to simplify your code with components that are contained within the hero class. By doing this, the hero class becomes more generic and this same pattern can then apply to any game object. When you decide to use composition to handle the various features, you’ll need to decide how the components will communicate with each other. One of the big goals of this design pattern is to keep the components independent of each other. How do you keep components independent but still allow communication? You can use the game object class to mediate between the components. Either directly by storing the result of one component so it can be used by another component or by managing messages that can be sent from one component through the game object class and then to all the other components. The messaging approach is much more complicated and may not always be needed. Or maybe you can choose multiple solutions and have some components behaving one or multiple ways. Common attributes that all game objects share can be updated directly. While less common attributes might be a good chance to use messages. If you’d like to read the book that describes this pattern along with diagrams and sample code, then you can find Game Programming Patterns at the Resources page. You can find all my favorite books and resources at this page to help you create better software designs. Listen to the full episode about this design pattern, or you can also read the full transcript below. Transcript The basic description says that this pattern allows an object type to span multiple domains without coupling between the domains. We’ve actually finished going through all the patterns in the Gang of Four book. There’s a really good book called Game Programming Patterns by Robert Nystrom that I recommend that documents several more patterns applicable to game development. This design pattern comes from this book. In order to understand this pattern, we’re going to have to dig a bit into the adventure hero class. In episode 78, I explained the state design pattern and how it could change how the hero behaved based on some state that could change. We’ll consider two states in this episode, walking and climbing a ladder. You also know about the game loop pattern from episode 83 and the update method pattern from episode 84. Let’s say we have a simple game loop that goes through input, update, and render operations in each frame. The game loop will also call into each game object instead of trying to do each of these three operations itself. Our hero class then will have similar methods that the game loop can call when needed. But how will the hero class implement these methods? A simple solution would look like this: The input method reads the keyboard or gamepad device to determine if a direction button is pressed. Or maybe the method pulls a command out of a queue if you’re following the command design pattern as described in episode 72. For this example, let’s say the up arrow is pressed to mean the hero should move forward. The input method then sets a velocity variable to show that the hero shou