87: Design Patterns: Event Queue.
Take Up Code - A podcast by Take Up Code: build your own computer games, apps, and robotics with podcasts and live classes
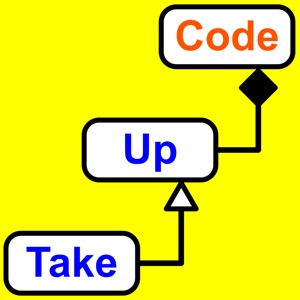
Categories:
What is the event queue design pattern? The event queue behavioral pattern allows you to keep track of work that needs to be done and let some other code actually perform the task. You get several benefits from the event queue design pattern: Code that has an interesting event doesn’t need to wait for the event to complete. It can just post an event and continue. Normally, events are handled on a first-in, first-out order but your code can change this if needed. The code that’s posting an event doesn’t need to worry about the order. An event queue decouples the sender of events from the handlers and allows each to proceed at their on pace. Event queues can be handled locally by a single object or can be distributed where many different objects use the queue to pull work from. If you’d like to read the book that describes this pattern along with diagrams and sample code, then you can find Game Programming Patterns at the Resources page. You can find all my favorite books and resources at this page to help you create better software designs. Listen to the full episode or you can read the full transcript below for more. Transcript The basic description says that this pattern stores a collection of either notifications or requests that will be handled in a first-in, first-out order. That’s the description from the book Game Programming Patterns and there’s a couple things I don’t agree with. By the way, if you read the book and compare it with my thoughts, you’ll gain even more insight. There are many things that I don’t explain from the book and things like this where I’ll recommend something different. First is the request part. An event queue isn’t a good choice of a pattern if you need to make a request. Usually, requests imply that you expect a reply. If your requests don’t need a reply, then okay, they might fit with this pattern. The second is the part about fist-in, first-out. This is usually called FIFO. Think of a line. I mean the kind where you wait in a line. Let’s say you’re at the post office and all the clerks are busy but nobody is waiting yet. You get in line and you’re the first in the line. While you’re waiting, another person arrives and gets in line after you. When a clerk becomes available at this time, who’s turn is it? Since you were the first in line, it’s your turn. This is a FIFO, or first-in, first-out. You probably wouldn’t be very happy if the line obeyed last-in, first out rules. If that was the case, you’d be stuck in that line all day as long as somebody else kept on arriving. Note that this is not cutting in line. That only happens in real life. Computers are normally too polite for stuff like that. But last-in, first-out queues are common in programming. They can also be called first-in, last out. It means the same thing, really. The reason I disagree about the order of the processing is because you could have an event queue that obeys either order. Or maybe even a hybrid where normally events are processed on a first-come, first-served approach while some events only get processed when the queue is empty. Alright, enough of the rambling. I haven’t even explained what this pattern is about yet and here I am discussing the finer points of queue orders. Imagine you work as a secretary for a busy manager and the manager walks over with a couple tasks that you need to perform. What does the manager do after letting you know about the tasks? Well, a smart manager will write each task separately on paper, or in separate emails, or in individual work orders however that sort of thing is tracked. Then after making sure you know about the tasks, the manager will continue with other things. In other words, the manager lines up the work you need to do. If another task comes along while you’re still working on the first one, then the new task gets recorded the same way. This