88: Design Patterns: Service Locator.
Take Up Code - A podcast by Take Up Code: build your own computer games, apps, and robotics with podcasts and live classes
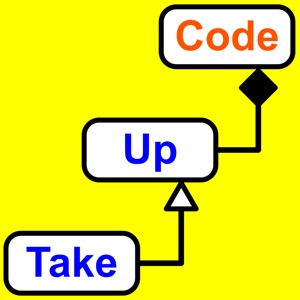
Categories:
What is the service locator design pattern? The service locator behavioral pattern allows you to make use of an interface without knowing what class implements the interface. This design pattern shares many aspects with the factory creational pattern. Both patterns allow you to use an interface without knowing exactly what class implements the interface. But while the factory design pattern is more focused on creating instances, this pattern is more focused on locating instances that already exist. Maybe you have some code that handles logging and you use this code throughout your application. You’re not going to want to pass a logging instance to every method you call so that means a lot of places in your code that will need some way to find a logger. Without this design pattern, you might consider making the logger a singleton. But this requires that your code know exactly which singleton to use to get to the logger. Instead of going directly to the logger, consider instead asking another class for the logger. This other class will be the service locator. And, yes, this means that you just traded one singleton for potentially another singleton. The point is though that your code is no longer coupled in all those places to a specific logger. It’s now coupled to a specific service locator. It uses the service locator to ask which logger it should use. This gives you the ability to easily swap one logger for another one. You’ll still have problems if you want to swap out one service locator for another one. You’ll probably want to avoid doing that. And asking another service locator for a service locator is also a bad idea. Just stick with a single service locator and let it help you manage the various services needed though your application. If you’d like to read the book that describes this pattern along with diagrams and sample code, then you can find Game Programming Patterns at the Resources page. You can find all my favorite books and resources at this page to help you create better software designs. Listen to the full episode about this design pattern, or you can also read the full transcript below. Transcript The basic description says that this pattern provides global access to a service without coupling your code to the concrete implementation. There’s a couple things that need explaining right away. First of all, what is a service and how do you use it? Well, a service can mean many things. Any code that can be called to perform some action or return some result can be thought of as a service. But that’s not what we normally think of as a service. A service is usually some useful and often unrelated functionality that your code needs. When I say unrelated, I don’t mean that the service has no meaning to your code. I just mean that the service provides some functionality whether your code calls it or not. You could have a number adder service that accepts two numbers, adds them for you, and returns the result. This would be rather pointless unless there was something special that the number adder service could provide. Maybe it could allow you to add really large numbers. Numbers to big for your platform to handle on its own. Now we’re getting somewhere. Sometimes we think of a service as a web service. This is functionality that you can access over a network. Usually the internet. The service will likely have access to special data such as map coordinates or weather information. A more common example though is logging. It’s very useful to be able to record events that happen while your program is running. Because if there are any problems, the log file can help a developer understand what might have went wrong. Logging is a great example for a service because it’s needed throughout your application and you need some way to get to it. Logging is interesting because it’s often included in your program as a library. This means the code exists and i